This exampe will use configuration by exception, which means the JPA Provider will map an entity to database using all the defaults.
Build
Create simple app using Maven, refer this post
Dependencies:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-validator</artifactId>
<version>5.3.0.Alpha1</version>
</dependency>
<dependency>
<groupId>org.eclipse.persistence</groupId>
<artifactId>org.eclipse.persistence.jpa</artifactId>
<version>2.6.4</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>6.0.5</version>
</dependency>
Create a POJO Class User,
package com.example;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.NamedQueries;
import javax.persistence.NamedQuery;
import javax.validation.constraints.NotNull;
import javax.validation.constraints.Size;
/**
* Created by zillani on 11/26/2016.
*/
@Entity
@NamedQueries({
@NamedQuery(name = "findAllUsers", query = "SELECT u FROM User u"),
@NamedQuery(name = "findUserByName", query = "SELECT u FROM User u WHERE u.username=:username")
})
public class User {
@Id
@GeneratedValue
private Long id;
@NotNull
private String username;
@NotNull
@Size(min = 6,max = 45)
private String password;
private String email;
private String phone;
private int age;
private String gender;
public User() {
}
public User(String username, String email) {
this.username = username;
this.email = email;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
}
Create persistence.xml file under resources/META-INF
<?xml version="1.0" encoding="UTF-8"?>
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd" version="2.1">
<persistence-unit name="simple-jpa" transaction-type="RESOURCE_LOCAL">
<provider>org.eclipse.persistence.jpa.PersistenceProvider</provider>
<class>com.example.User</class>
<properties>
<property name="javax.persistence.schema-generation.database.action" value="drop-and-create"/>
<property name="javax.persistence.jdbc.driver" value="com.mysql.cj.jdbc.Driver"/>
<property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/test"/>
<property name="javax.persistence.jdbc.user" value="root"/>
<property name="javax.persistence.jdbc.password" value="root"/>
<property name="javax.persistence.sql-load-script-source" value="insert.sql"/>
<property name="eclipselink.logging.level" value="INFO"/>
</properties>
</persistence-unit>
</persistence>
Create sql file under resources
INSERT INTO test.user(ID,USERNAME,PASSWORD,EMAIL,PHONE,GENDER,AGE)VALUES(2,"zillani","root","shaikzillani@gmail.com","9247411984","male",25);
INSERT INTO test.user(ID,USERNAME,PASSWORD,EMAIL,PHONE,GENDER,AGE)VALUES(3,"Humayun","root","humayunsyed@gmail.com","9991234812","male",23);
INSERT INTO test.user(ID,USERNAME,PASSWORD,EMAIL,PHONE,GENDER,AGE)VALUES(4,"Mahesh","root","mahesh.b@gmail.com","9562235658","male",25);
Create a executable class, Main
package com.example;
import javax.persistence.EntityManager;
import javax.persistence.EntityManagerFactory;
import javax.persistence.EntityTransaction;
import javax.persistence.Persistence;
import java.util.List;
public class Main {
public static void main(String[] args) {
User user = new User();
user.setAge(25);
user.setEmail("stranger@gmail.com");
user.setGender("female");
user.setPassword("root");
user.setPhone("9948184406");
user.setUsername("rh");
EntityManagerFactory entityManagerFactory = Persistence.createEntityManagerFactory("simple-jpa");
EntityManager entityManager = entityManagerFactory.createEntityManager();
EntityTransaction entityTransaction = entityManager.getTransaction();
//INSERT USER INTO DB
entityTransaction.begin();
entityManager.persist(user);
entityTransaction.commit();
// FIND ALL USERS
List<User> allUsers = entityManager.createNamedQuery("findAllUsers",User.class).getResultList();
System.out.println("############## ALL USERS ###################");
for (User allUser : allUsers) {
System.out.println(allUser.getUsername());
}
System.out.println("############## ALL USERS ###################");
//FIND USER BY USERNAME
String un = "zillani";
User foundUser = entityManager.createNamedQuery("findUserByName",User.class).setParameter("username",un).getSingleResult();
System.out.println("################ FIND USER BY USERNAME #################");
System.out.println(foundUser.getUsername());
System.out.println("################ FIND USER BY USERNAME #################");
entityManager.close();
entityManagerFactory.close();
}
}
The Following should be the project structure, build the project using mvn clean install,
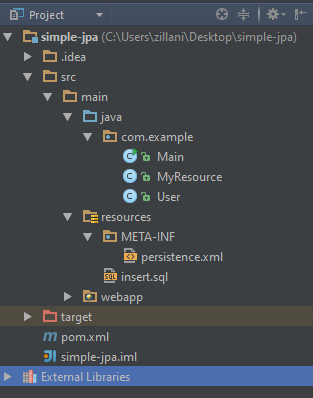
Running the main class should insert new Users into the database and verify the retrieved.
Download the example here